LeNet-5 卷积神经网络的 4 种实现方法
LeNet-5 是 LeCun 在 1998 年发明的卷积神经网络 ↗ ,其结构简单,很适合初学者用来学习卷积神经网络的构建方法。
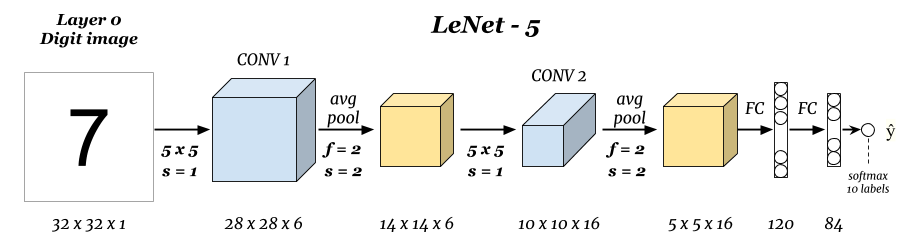
下面,我们将使用 TensorFlow 和 PyTorch 提供的低阶和高阶 API 来构建 LeNet-5 网络,总共会用到 4 种不同的方法。
TensorFlow 低阶 API 构建
TensorFlow 低阶 API 主要是利用 tf.nn
模块提供的相关函数或类方法。特别注意的是,你需要自行初始化卷积核权重和全连接层的截距项。
import tensorflow as tf
def LeNet(x):
# 卷积核权重
weights = {
'conv1': tf.Variable(tf.random_normal(shape=(5, 5, 1, 6))),
'conv2': tf.Variable(tf.random_normal(shape=(5, 5, 6, 16))),
'fc1': tf.Variable(tf.random_normal(shape=(5*5*16, 120))),
'fc2': tf.Variable(tf.random_normal(shape=(120, 84))),
'out': tf.Variable(tf.random_normal(shape=(84, 10))),
}
# 卷积层 1: Input = 32x32x1. Output = 28x28x6.
conv1 = tf.nn.conv2d(x, weights['conv1'], strides=[1, 1, 1, 1], padding='VALID')
# RELU 激活
conv1 = tf.nn.relu(conv1)
# 池化层 1: Input = 28x28x6. Output = 14x14x6.
pool1 = tf.nn.avg_pool(conv1, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='VALID')
# 卷积层 2: Input = 14x14x6. Output = 10x10x16.
conv2 = tf.nn.conv2d(pool1, weights['conv2'], strides=[1, 1, 1, 1], padding='VALID')
# RELU 激活
conv2 = tf.nn.relu(conv2)
# 池化层 2: Input = 10x10x16. Output = 5x5x16.
pool2 = tf.nn.avg_pool(conv2, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='VALID')
# 展平. Input = 5x5x16. Output = 400.
flatten = tf.reshape(pool2, [-1, 5*5*16])
# 截距项
biases = {
'fc1': tf.Variable(tf.zeros(120)),
'fc2': tf.Variable(tf.zeros(84)),
'out': tf.Variable(tf.zeros(10))
}
# 全连接层
fc1 = tf.nn.relu(tf.add(tf.matmul(flatten, weights['fc1']), biases['fc1']))
fc2 = tf.nn.relu(tf.add(tf.matmul(fc1, weights['fc2']), biases['fc2']))
outs = tf.add(tf.matmul(fc2, weights['out']), biases['out'])
return outs
LeNet-5 要求的传入数据尺寸是 [32, 32, 1]
,所以像常用的 MNIST 等数据集都需要预先处理成规定的形状才能使用该网络函数。
TensorFlow 高阶 API 构建
目前,Keras 已正式合并入 TensorFlow。所以 TensorFlow 的高阶 API 及可以通过 tf.keras
来构建。我们这里使用序贯模型结构,很方便地搭建出 LeNet-5 网络。这也是最简单的方法之一。
import tensorflow as tf
model = tf.keras.Sequential() # 构建序贯模型
# 卷积层,6 个 5x5 卷积核,步长为 1,relu 激活,第一层需指定 input_shape
model.add(tf.keras.layers.Conv2D(filters=6, kernel_size=(5, 5), strides=(1, 1),
activation='relu', input_shape=(32, 32, 1)))
# 平均池化,池化窗口默认为 2
model.add(tf.keras.layers.AveragePooling2D(pool_size=(2, 2), strides=2))
# 卷积层,16 个 5x5 卷积核,步为 1,relu 激活
model.add(tf.keras.layers.Conv2D(filters=16, kernel_size=(
5, 5), strides=(1, 1), activation='relu'))
# 平均池化,池化窗口默认为 2
model.add(tf.keras.layers.AveragePooling2D(pool_size=(2, 2), strides=2))
# 需展平后才能与全连接层相连
model.add(tf.keras.layers.Flatten())
# 全连接层,输出为 120,relu 激活
model.add(tf.keras.layers.Dense(units=120, activation='relu'))
# 全连接层,输出为 84,relu 激活
model.add(tf.keras.layers.Dense(units=84, activation='relu'))
# 全连接层,输出为 10,Softmax 激活
model.add(tf.keras.layers.Dense(units=10, activation='softmax'))
# 查看网络结构
model.summary()
除此之外,你还可以使用 TensorFlow 提供的 Estimator 高阶 API 来构建,这里不再赘述。详细可参阅 官方文档。
PyTorch 低阶 API 构建
相比于 TensorFlow,PyTorch 拥有无需管理会话,自动求导等机制,越来越多的研究人员偏好使用。使用 PyTorch 实现 LeNet-5 时,需要继承 nn.Module
基类,然后使用 torch.nn
提供的类或 torch.nn.functional
提供的函数来组合模型结构。
import torch
import torch.nn as nn
import torch.nn.functional as F
class LeNet(nn.Module):
def __init__(self):
super(LeNet, self).__init__()
# 卷积层 1
self.conv1 = nn.Conv2d(
in_channels=1, out_channels=6, kernel_size=(5, 5), stride=1)
# 池化层 1
self.pool1 = nn.AvgPool2d(kernel_size=(2, 2))
# 卷积层 2
self.conv2 = nn.Conv2d(
in_channels=6, out_channels=16, kernel_size=(5, 5), stride=1)
# 池化层 2
self.pool2 = nn.AvgPool2d(kernel_size=(2, 2))
# 全连接层
self.fc1 = nn.Linear(in_features=5*5*16, out_features=120)
self.fc2 = nn.Linear(in_features=120, out_features=84)
self.fc3 = nn.Linear(in_features=84, out_features=10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = self.pool1(x)
x = F.relu(self.conv2(x))
x = self.pool2(x)
x = x.reshape(-1, 5*5*16)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = F.softmax(self.fc3(x), dim=1)
return x
PyTorch 高阶 API 构建
实际上,PyTorch 也提供了 nn.Sequential
容器结构,类似于 Keras 的使用过程。nn.Sequential
可以简化模型的构建过程,直接使用 torch.nn
提供的类来组合即可。注意,PyTorch 中未提供 Flatten 类,所以需要使用 reshape
操作预先定义一个。
import torch.nn as nn
class Flatten(nn.Module):
def forward(self, input):
return input.reshape(input.size(0), -1)
# 构建 Sequential 容器结构
model = nn.Sequential(
nn.Conv2d(1, 6, (5, 5), 1),
nn.ReLU(),
nn.AvgPool2d((2, 2)),
nn.Conv2d(6, 16, (5, 5), 1),
nn.ReLU(),
nn.AvgPool2d((2, 2)),
Flatten(),
nn.Linear(5*5*16, 120),
nn.ReLU(),
nn.Linear(120, 84),
nn.ReLU(),
nn.Linear(84, 10),
nn.Softmax(dim=1)
)
上面即是如何使用 TensorFlow 和 PyTorch 实现经典卷积神经网络 LeNet-5 的 4 种方法。当然,这里只搭建了模型的主体结构,如果要正常开始训练,还需要对数据预处理或补充模型训练和测试代码。更多的细节内容,可以结合框架的官方文档来实现。